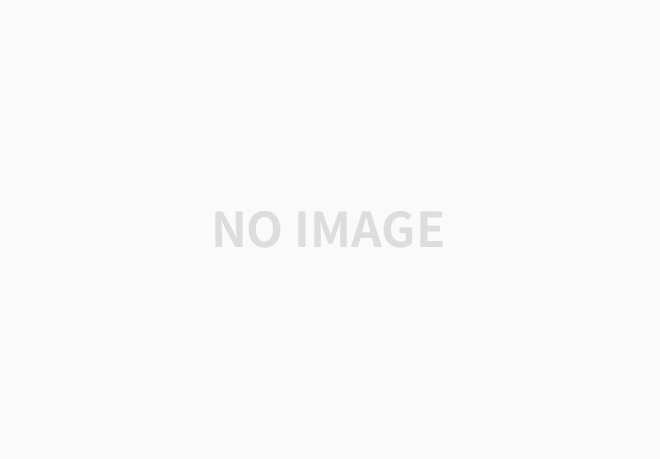
문제 풀이 정리
Find Peak Element
A peak element is an element that is strictly greater than its neighbors.
Given an integer array nums, find a peak element, and return its index. If the array contains multiple peaks, return the index to any of the peaks.
You may imagine that nums[-1] = nums[n] = -∞.
You must write an algorithm that runs in O(log n) time.
Example 1:
Input: nums = [1,2,3,1]
Output: 2
Explanation: 3 is a peak element and your function should return the index number 2.
Example 2:
Input: nums = [1,2,1,3,5,6,4]
Output: 5
Explanation: Your function can return either index number 1 where the peak element is 2, or index number 5 where the peak element is 6.
Constraints:
- 1 <= nums.length <= 1000
- -2^31 <= nums[i] <= 2^31 - 1
- nums[i] != nums[i + 1] for all valid i.
코드
class Solution:
def findPeakElement(self, nums: List[int]) -> int:
left = 0
right = len(nums)-1
while left <= right:
if left == right:
return left
if left > 0 and right < len(nums)-1:
if nums[left] > nums[left-1] and nums[left] > nums[left+1]:
return left
else:
left += 1
if nums[right] > nums[right-1] and nums[right] > nums[right+1]:
return right
else:
right -= 1
else:
if left == 0 and nums[left] > nums[left+1]:
return left
else:
left += 1
if right == len(nums)-1 and nums[right] > nums[right-1]:
return right
else:
right -= 1
문제 넋두리
(한번 쓰다가 다 날아가서 다시쓴다..ㅂㄷㅂㄷ)
양옆의 숫자보다 큰 숫자를 찾는 문제이다.
단순하게 생각하면 배열의 값중 제일 큰 숫자를 찾는 문제일수 있으나, 문제에 적힌 O(logN) 으로 풀어야 된다고 해서 정렬은 탈락
정렬을 안하고도 찾을 수 있지 않을까? 해서 이진트리를 이용하자 싶었다.
mid = (left + right) // 2 형식으로 left, right 를 움직이며 찾아보려 했지만 계속 되는 실패...
더이상 좋은 수가 떠오르지 않아 포기하고 구글을 검색했다.
구글에서 찾은 바는 너무나 간단했다.
배열의 양끝부터 하나씩 움직이며 양옆의 값보다 큰 값을 찾으면 된다...
이럴수가.. 이렇게 쉬운 풀이가 있다니...
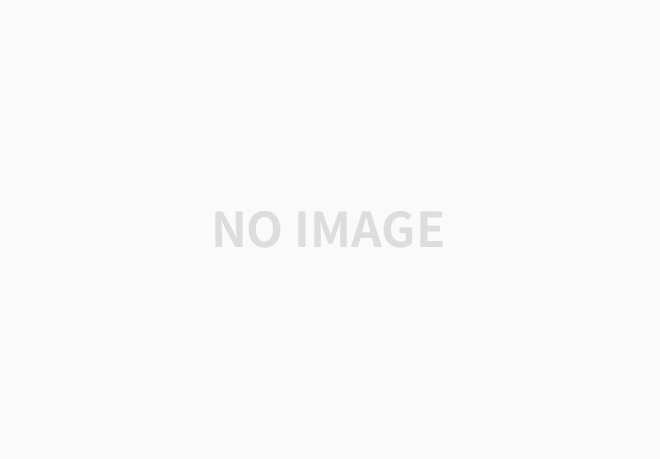
'algorithm > leetcode' 카테고리의 다른 글
Intersection of Two Arrays II [leetcode] (0) | 2021.09.18 |
---|---|
Reverse Nodes in k-Group [LeetCode] (0) | 2021.07.18 |
Isomorphic Strings [LeetCode] (0) | 2021.07.12 |
Find Median from Data Stream [LeetCode] (0) | 2021.07.12 |
Reduce Array Size to The Half [LeetCode] (0) | 2021.07.07 |
댓글